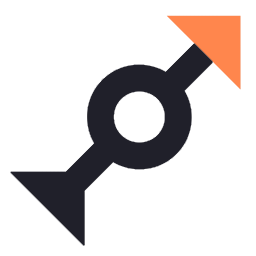
Middleware
Middleware plays a crucial role in state management, particularly in libraries like Redux. It acts as an intermediary layer between the action dispatch and the reducer, allowing developers to enhance and extend the functionality of their state management system.
How Middleware Works
Middleware intercepts actions before they reach the reducer, enabling developers to perform additional tasks or modify the action itself. Here’s a breakdown of how middleware functions:
-
Interception: When an action is dispatched, it first passes through the middleware chain before reaching the reducer[1][2].
-
Processing: Each middleware in the chain can access the dispatched action, the current state, and the
dispatch
function[2]. -
Modification: Middleware can modify actions, dispatch new actions, or even cancel actions entirely[1][5].
-
Chaining: Multiple middleware can be combined, with each passing the action to the next in the chain using the
next
function[7].
Common Use Cases
Middleware is particularly useful for handling various aspects of state management:
-
Asynchronous Operations: Middleware like Redux Thunk allows action creators to return functions instead of plain objects, enabling asynchronous operations such as API calls[10].
-
Logging: Middleware can log actions and state changes, which is invaluable for debugging and monitoring application behavior[2][5].
-
Error Handling: Middleware can catch and process errors before they reach the reducer, enhancing application stability[1].
-
Authentication and Authorization: Middleware can intercept actions related to protected routes or sensitive data, enforcing security rules[2].
-
Caching: Middleware can implement caching mechanisms, intercepting actions and serving cached data when appropriate[2].
Implementation Example
Here’s a simple example of a logging middleware:
const loggerMiddleware = (store) => (next) => (action) => {
console.log('Dispatching:', action);
console.log('Current State:', store.getState());
const result = next(action);
console.log('Updated State:', store.getState());
return result;
};
This middleware logs the action being dispatched, the current state before the action is processed, and the updated state after the action is processed[2].
Benefits of Using Middleware
-
Separation of Concerns: Middleware allows developers to extract complex logic from components and action creators, leading to cleaner, more maintainable code[1][5].
-
Reusability: Common functionalities can be encapsulated in middleware and reused across different parts of the application[8].
-
Extensibility: Middleware provides a flexible way to extend the capabilities of state management systems without modifying their core logic[5].
By leveraging middleware, developers can create more robust, efficient, and feature-rich applications while maintaining a clean and organized codebase.
References
- [1] https://www.linkedin.com/pulse/understanding-middleware-react-enhancing-application-olga-green
- [2] https://www.linkedin.com/pulse/middleware-redux-enhancing-your-state-management-olga-green
- [3] https://nextjs.org/docs/pages/building-your-application/routing/middleware
- [4] https://stackoverflow.com/questions/51384931/accessing-state-in-middleware-in-redux
- [5] https://mobileappcircular.com/understanding-middleware-in-redux-a-comprehensive-guide-cc161a0cfcf?gi=fa973befd708
- [6] https://azure.microsoft.com/en-us/resources/cloud-computing-dictionary/what-is-middleware
- [7] https://redux.js.org/understanding/history-and-design/middleware
- [8] https://vocal.media/journal/demystifying-middleware-in-react-a-comprehensive-guide
- [9] https://www.geeksforgeeks.org/what-are-middlewares-in-react-redux/
- [10] https://www.geeksforgeeks.org/what-is-the-use-of-middleware-redux-thunk/
- [11] https://aws.amazon.com/what-is/middleware/
- [12] https://www.reddit.com/r/reactjs/comments/1anza21/when_to_use_state_management_libraries/