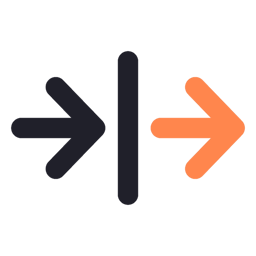
Proxy
Using a proxy in frontend development offers several benefits, including improved security, performance optimization, and simplified API interactions. Here’s how to implement and use a proxy in your frontend application:
Setting Up a Proxy
1. Create React App
For Create React App projects, you can easily set up a proxy by adding a “proxy” field to your package.json file:
{
"proxy": "http://localhost:4000"
}
This will forward any unknown requests to the specified URL[26].
2. Manual Configuration
For more control, you can manually configure the proxy:
Install the http-proxy-middleware package:
npm install http-proxy-middleware --save
Create a setupProxy.js file in your src directory:
const { createProxyMiddleware } = require('http-proxy-middleware');
module.exports = function(app) {
app.use(
'/api',
createProxyMiddleware({
target: 'http://localhost:5000',
changeOrigin: true,
})
);
};
This setup will proxy any request to /api to your backend server[26].
Benefits of Using a Proxy
-
Security Enhancement: Proxies act as a barrier between clients and servers, filtering malicious traffic and protecting against attacks[4].
-
Performance Optimization: Proxies can cache frequently accessed content, reducing bandwidth usage and improving load times[4].
-
Simplified API Interaction: Proxies can abstract complex API interactions, making it easier for developers to work with backend services[32].
-
Cross-Origin Resource Sharing (CORS) Mitigation: Proxies help bypass CORS restrictions during development, simplifying API requests[18].
-
Load Balancing: In production environments, proxies can distribute traffic across multiple backend servers, improving overall system performance[4].
Best Practices
-
Security First: Always use HTTPS for production proxies to ensure encrypted data transfer[27].
-
Careful Configuration: Be mindful of your proxy settings, especially when dealing with sensitive data or authentication[27].
-
Monitoring and Logging: Implement proper logging and monitoring for your proxy to track usage and identify potential issues[32].
-
Environment-Specific Configuration: Use different proxy configurations for development and production environments[26].
By implementing a proxy in your frontend application, you can create a more secure, efficient, and developer-friendly environment for your web development projects.
References
- [1] https://floqast.com/engineering-blog/post/lightweight-microfrontend-development-with-a-local-proxy-server/
- [2] https://www.youtube.com/watch?v=qfYC-K-DKRQ
- [3] https://docs.precisely.services/docs/sftw/spectrum/22.1/en/webhelp/Spatial/Spatial/source/Development/devguide/ria/proxy/usingriaproxy.html
- [4] https://blogs.halodoc.io/proxy-servers-web-developer-perspective/
- [5] https://www.geeksforgeeks.org/proxy-servers-advantages/
- [6] https://thesocialproxy.com/2023/06/23/proxies-in-web-development/
- [7] https://www.geeksforgeeks.org/how-proxy-backend-server-using-react-js/
- [8] https://www.hostwinds.com/blog/what-is-a-proxy-server-types-uses-pros-cons-more
- [9] https://www.varonis.com/blog/what-is-a-proxy-server
- [10] https://www.cerberusftp.com/blog/four-benefits-of-using-a-reverse-proxy-server/
- [11] https://stackoverflow.com/questions/76697837/what-is-use-of-proxy-in-frontend-part
- [12] https://blog.kronis.dev/tutorials/how-to-use-nginx-to-proxy-your-front-end-and-back-end
- [13] https://security.stackexchange.com/questions/187074/making-websites-use-proxies
- [14] https://docs.sunbirdrc.dev/use/developers-guide/configuration/frontend-proxy-configuration
- [15] https://stackoverflow.com/questions/66774222/how-can-i-set-up-a-proxy-for-an-unowned-site-to-test-front-end-changes
- [16] https://learn.microsoft.com/en-us/windows-server/remote/remote-access/web-application-proxy/web-app-proxy-windows-server
- [17] https://www.reddit.com/r/node/comments/upk3dj/what_is_reverse_proxy_and_how_i_can_learn_it/
- [18] https://blog.logrocket.com/why-you-should-use-proxy-server-create-react-app/
- [19] https://www.fortinet.com/resources/cyberglossary/reverse-proxy
- [20] https://dribbble.com/stories/2023/03/28/why-web-designers-should-use-a-proxy
- [21] https://konghq.com/blog/engineering/api-gateway-vs-api-proxy-understanding-the-differences
- [22] https://serverfault.com/questions/25071/will-a-reverse-proxy-in-front-of-web-server-improve-security/25095
- [23] https://stackoverflow.com/questions/6763571/advantages-of-a-reverse-proxy-in-front-of-node-js/56302977
- [24] https://www.haproxy.com/documentation/haproxy-configuration-tutorials/core-concepts/frontends/
- [25] https://www.geeksforgeeks.org/web-server-proxies-and-their-role-in-designing-systems/
- [26] https://create-react-app.dev/docs/proxying-api-requests-in-development/
- [27] https://www.theserverside.com/blog/Coffee-Talk-Java-News-Stories-and-Opinions/How-to-setup-Nginx-reverse-proxy-servers-by-example
- [28] https://www.ramotion.com/blog/web-app-proxy/
- [29] https://clerk.com/docs/advanced-usage/using-proxies
- [30] https://forum.proxmox.com/threads/using-nginx-as-reverse-proxy-externally.116127/
- [31] https://learn.microsoft.com/en-us/previous-versions/windows/it-pro/windows-server-2012-r2-and-2012/dn584113(v=ws.11)
- [32] https://blog.dreamfactory.com/when-to-use-an-api-proxy-over-an-api-gateway
- [33] https://themewagon.com/blog/using-proxies-in-web-development/
- [34] https://www.geeksforgeeks.org/why-should-we-use-proxy-server/
- [35] https://cyclr.com/resources/developer/what-is-an-api-proxy-and-how-does-it-work
- [36] https://www.strongdm.com/blog/difference-between-proxy-and-reverse-proxy