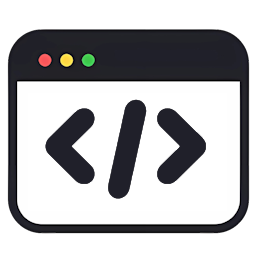
Protocol and Security
This document covers key protocols and technologies for securing web applications and improving user experience. The following topics are covered:
- Service Worker & Progressive Web Apps (PWA)
- The
.well-known
Directory for SSL/TLS - Self-Signed Certificates (using
mkcert
) - Let’s Encrypt SSL Certificates
- Overview of Internet Protocols
1. Service Worker & Progressive Web Apps (PWA)
A Service Worker is a script that runs in the background of a web application, allowing it to control caching, enable offline functionality, and handle background sync. When combined with Progressive Web Apps (PWA) technologies, service workers enhance the web app experience by enabling offline access, push notifications, and faster load times.
Service Worker with Secure Connection
Service Workers require the application to be served over HTTPS (with the exception of localhost), as they can intercept network requests and manipulate responses, making them powerful but potentially dangerous if used over an insecure connection. Secure connections ensure that sensitive data remains protected.
MDN’s Cycle Tracker Tutorial - Service Worker Example
In the MDN tutorial for CycleTracker, the service worker is used to cache assets and handle the application offline. The web app needs to be served over HTTPS, and the following is a simplified version of setting up a service worker:
Steps:
- Create the Service Worker:
In the root directory, create
service-worker.js
with the necessary caching logic.self.addEventListener('install', event => { event.waitUntil( caches.open('my-cache').then(cache => { return cache.addAll([ '/', '/index.html', '/styles.css', '/app.js' ]); }) ); }); self.addEventListener('fetch', event => { event.respondWith( caches.match(event.request).then(response => { return response || fetch(event.request); }) ); });
- Register the Service Worker:
In your main JavaScript file (e.g.,
app.js
), register the service worker:if ('serviceWorker' in navigator) { navigator.serviceWorker.register('/service-worker.js').then(registration => { console.log('Service Worker registered with scope: ', registration.scope); }).catch(error => { console.log('Service Worker registration failed: ', error); }); }
-
HTTPS Requirement: The application must be served over HTTPS. Modern browsers block service workers on insecure sites (non-HTTPS).
- Testing: Test the functionality by disconnecting from the network and observing that the PWA still functions (i.e., cached content is available).
2. The .well-known
Directory for SSL/TLS
The .well-known
directory is a hidden folder located at the root of a website’s domain and contains standardized configuration files that are used by various protocols. It is commonly used for exposing public keys, verification tokens, or configuration files needed for security protocols like SSL/TLS.
Key Uses:
- ACME Challenge for SSL Certificates: Tools like Let’s Encrypt use the
.well-known/acme-challenge
directory for domain verification during SSL certificate issuance. - Security Policies: Hosting files like HTTP Public Key Pinning (HPKP) or Content Security Policy (CSP) headers in the
.well-known
directory.
Example Path:
- For ACME Challenge, the URL path for verification looks like:
https://yourdomain.com/.well-known/acme-challenge/<token>
Example (ACME Challenge):
- ACME Challenge File: The server must serve a file from
.well-known/acme-challenge/
to verify domain ownership. - Let’s Encrypt or other Certificate Authorities (CAs) will use this file to validate the request for an SSL certificate.
3. Self-Signed SSL Certificates (mkcert
)
A self-signed certificate is an SSL certificate that is signed by the entity that created it rather than a trusted certificate authority (CA). While these certificates are useful for local development, they are not trusted by browsers for production environments.
Using mkcert
for Self-Signed Certificates
mkcert
is a simple tool that helps you create locally trusted self-signed certificates. It adds the root CA to your system’s trust store, making the certificates created by mkcert
trusted by browsers.
Steps to Use mkcert
:
- Install mkcert:
For macOS:
brew install mkcert
For Windows/Linux (via Chocolatey or similar package managers):
choco install mkcert
- Create a Local CA (Certificate Authority):
Run the following command to set up a local certificate authority:
mkcert -install
- Generate a Certificate for Your Local Domain:
For example, to generate a certificate for
localhost
:mkcert localhost
This will generate two files:
localhost.pem
(certificate) andlocalhost-key.pem
(private key). -
Configure Your Local Server: Use the generated certificate and private key in your local development server, e.g., with Node.js or Apache.
- Testing:
Your browser will now trust the self-signed certificate when accessing
https://localhost
.
4. Let’s Encrypt SSL Certificates
Let’s Encrypt is a free, automated, and open Certificate Authority (CA) that provides SSL certificates to secure websites. It simplifies the process of obtaining and renewing certificates via the ACME protocol.
Steps to Use Let’s Encrypt with Certbot
-
Install Certbot on Ubuntu:
On Ubuntu, the easiest way to install Certbot is using the
apt
package manager:sudo apt update sudo apt install certbot python3-certbot-apache
- Obtain an SSL Certificate:
After installing Certbot, run the following command to obtain an SSL certificate for your domain (replace
yourdomain.com
with your actual domain):sudo certbot --apache -d yourdomain.com -d www.yourdomain.com
Certbot will automatically configure your Apache server to use HTTPS.
- Auto-Renewal:
Let’s Encrypt certificates are valid for 90 days. You can set up a cron job to automatically renew them:
sudo crontab -e
Add this line to renew the certificate automatically:
0 0 * * * certbot renew --quiet
- Verify Renewal:
To verify that your certificates are renewed correctly, run:
sudo certbot renew --dry-run
- Testing:
After setting up, visit
https://yourdomain.com
in the browser to confirm that the SSL certificate is working.
5. Internet Protocols Overview
The Internet Protocols encompass the set of rules that define how data is transmitted and received over the internet. Below are the common types of protocols:
- Transmission Control Protocol (TCP): Ensures reliable transmission of data packets between systems, and provides error checking and correction.
- Hypertext Transfer Protocol (HTTP/HTTPS): The protocol used for transferring web pages. HTTPS is the secure version, using SSL/TLS for encryption.
- File Transfer Protocol (FTP): Used for transferring files over a network. FTP operates in both active and passive modes.
- Domain Name System (DNS): Resolves domain names (like
example.com
) to IP addresses, allowing browsers to connect to the correct web servers. - Simple Mail Transfer Protocol (SMTP): A protocol for sending emails between servers.
- Post Office Protocol (POP3): Used by email clients to retrieve emails from a server.
- Internet Message Access Protocol (IMAP): Another protocol for retrieving emails from a server, with more advanced features compared to POP3.
- Secure Shell (SSH): Provides a secure command-line interface for remote administration of servers.
- Virtual Private Network (VPN): Allows for secure remote access to a network through encryption.
Protocols and Their Applications:
- HTTP/HTTPS: Browsing websites.
- FTP: File uploads/downloads (used by developers and website admins).
- DNS: Ensuring correct IP address resolution.
- SMTP/POP3/IMAP: Email communication.
References
- MDN Service Workers
- .wellknown
- Lets Encrypt
- https://gist.github.com/Grawl/bd0096b49276934c807b4a74088b081c
- https://www.geeksforgeeks.org/types-of-internet-protocols/#